In the earlier
tutorial we discussed how to setup AWS IOT for an Amazon user account. Now lets see how to use the Arduino esp32 AWS IOT library to communicate with AWS server.
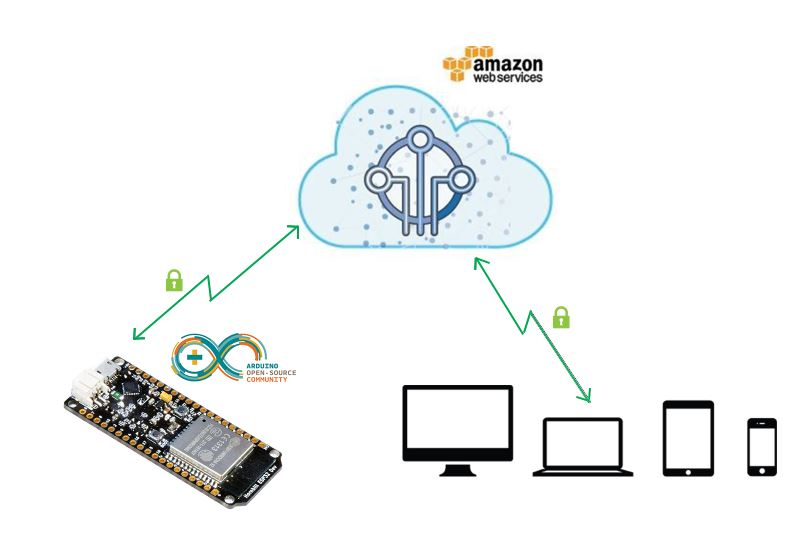
AWS IOT for ESP32
Download the AWS IOT library for Arduino ESP32 from
this link.
Now open the first example pubSubTest.ino as shown below.
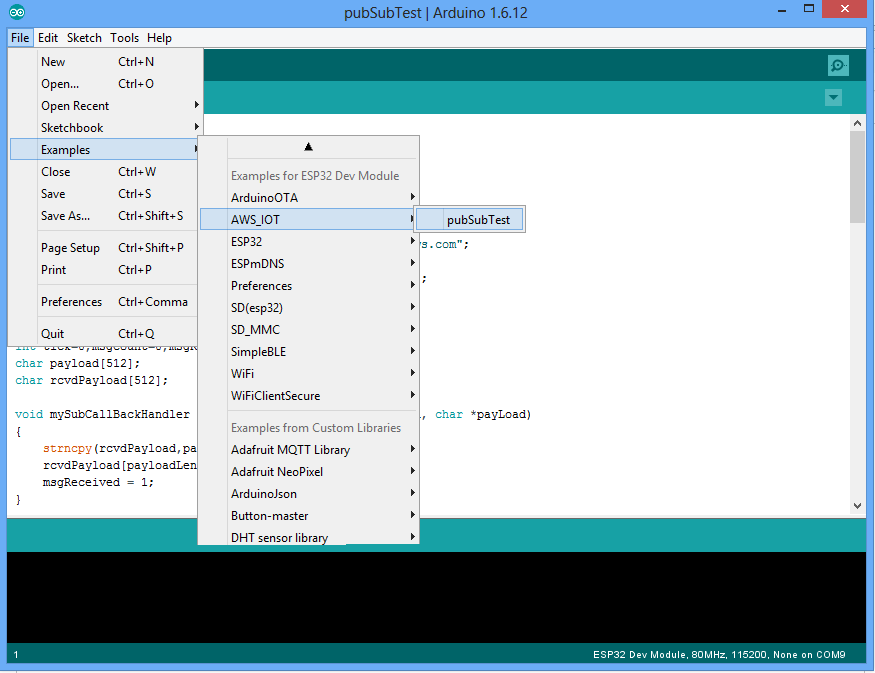
.
Wifi SSID and Host Address Configuration
Configure the below parameters at the beginning of the sketch as shown in the image.
WIFI_SSID
WIFI_PASSWORD
HOST_ADDRESS
CLIENT_ID
TOPIC_NAME
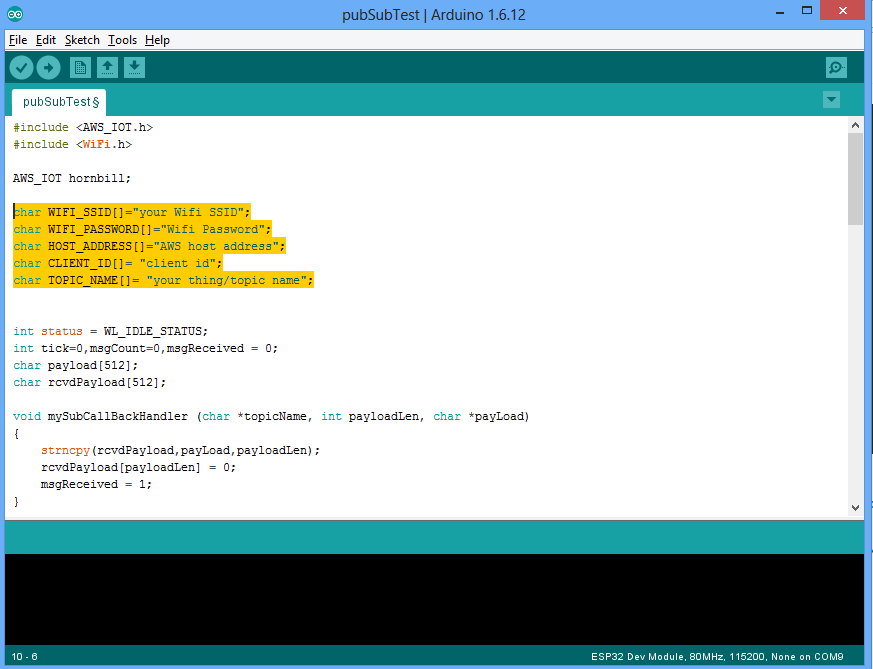
AWS Certificate Configuration
The below certificate and private key needs to be copied to aws_iot_certificate.c file in the form of array.
- aws-root-ca.pem
- certificate.pem.crt
- private.pem.key
Upload the sketch and verify the output
Publish and subscribe(received) messages printed on terminal.
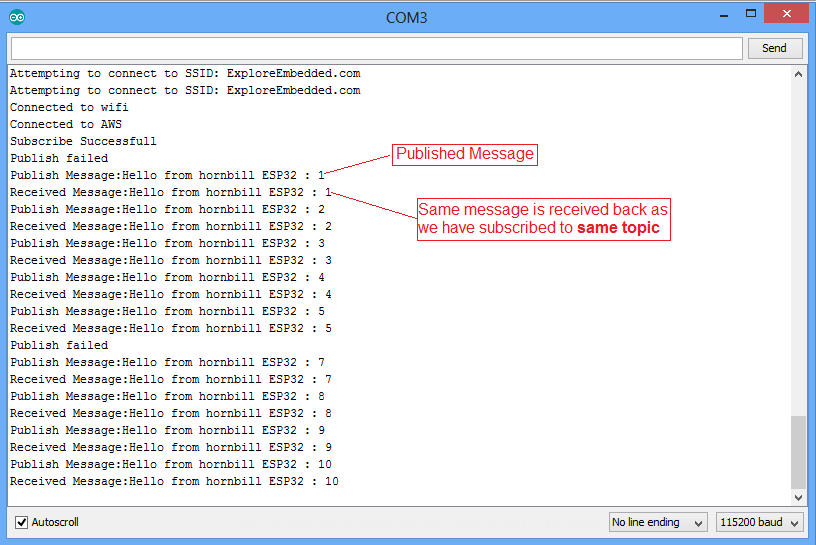
Published message viewed on MQTT Fx client software:
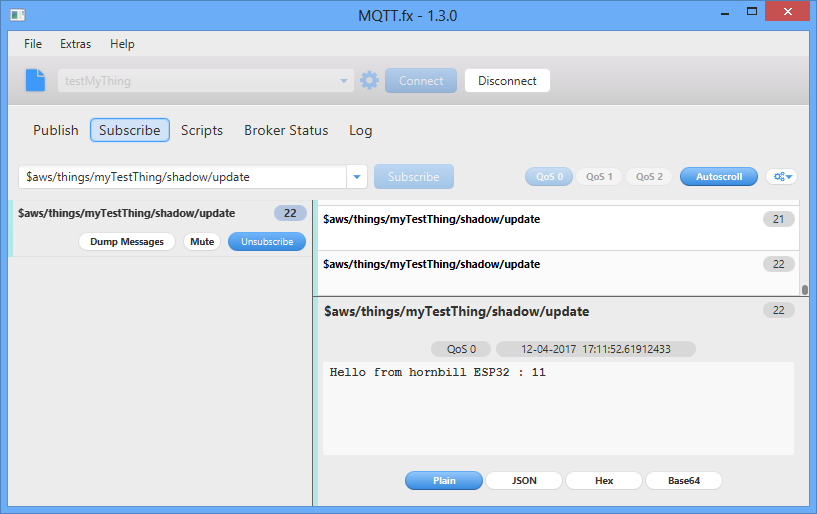
Publish a message from MQTT Fx Client and verify the received message on terminal.
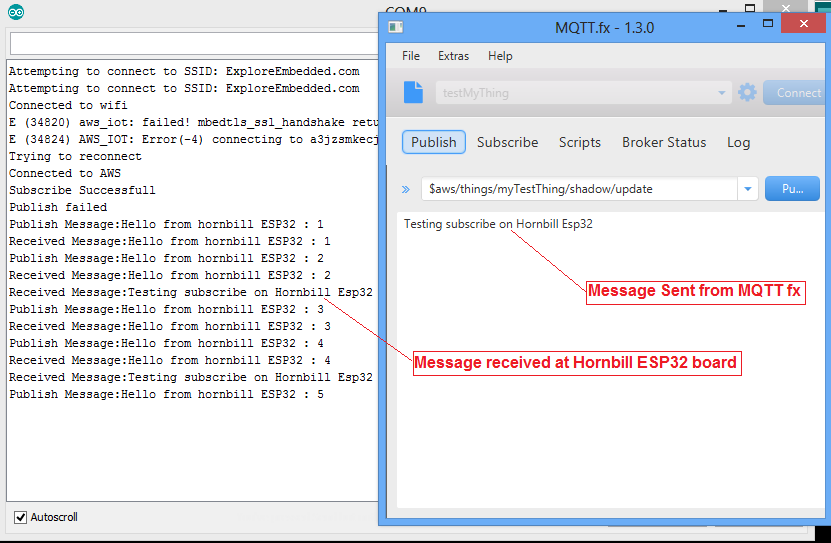
Logging Temperature and Humidity using DHT11 sensor
After setting the AWS client and AWS IOT lib, lets log the real time temperature and humidity using DHT11 sensor.
Install the Adafruit DHT11 sensor library using Arduino Library Manager or download it from
this link.
Do the connection as shown below.
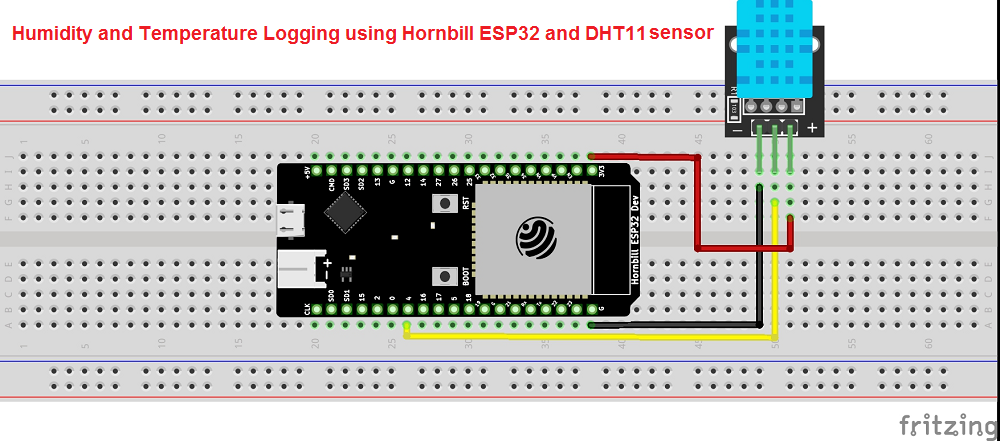
Now copy paste the below code and configure the Wifi SSID, Host Address and upload the sketch.
|
|
| #include <AWS_IOT.h> |
| #include <WiFi.h> |
|
|
| #include "DHT.h" |
|
|
| #define DHTPIN 4 // what digital pin we're connected to |
|
|
| // Uncomment whatever type you're using! |
| #define DHTTYPE DHT11 // DHT 11 |
| //#define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321 |
| //#define DHTTYPE DHT21 // DHT 21 (AM2301) |
|
|
| DHT dht(DHTPIN, DHTTYPE); |
|
|
| AWS_IOT hornbill; // AWS_IOT instance |
|
|
| char WIFI_SSID[]="your Wifi SSID"; |
| char WIFI_PASSWORD[]="Wifi Password"; |
| char HOST_ADDRESS[]="AWS host address"; |
| char CLIENT_ID[]= "client id"; |
| char TOPIC_NAME[]= "your thing/topic name"; |
|
|
|
|
| int status = WL_IDLE_STATUS; |
| int tick=0,msgCount=0,msgReceived = 0; |
| char payload[512]; |
| char rcvdPayload[512]; |
|
|
|
|
| void setup() { |
| |
| Serial.begin(115200); |
| delay(2000); |
|
|
| while (status != WL_CONNECTED) |
| { |
| Serial.print("Attempting to connect to SSID: "); |
| Serial.println(WIFI_SSID); |
| // Connect to WPA/WPA2 network. Change this line if using open or WEP network: |
| status = WiFi.begin(WIFI_SSID, WIFI_PASSWORD); |
|
|
| // wait 5 seconds for connection: |
| delay(5000); |
| } |
|
|
| Serial.println("Connected to wifi"); |
|
|
| if(hornbill.connect(HOST_ADDRESS,CLIENT_ID)== 0) // Connect to AWS using Host Address and Cliend ID |
| { |
| Serial.println("Connected to AWS"); |
| delay(1000); |
| } |
| else |
| { |
| Serial.println("AWS connection failed, Check the HOST Address"); |
| while(1); |
| } |
|
|
| delay(2000); |
|
|
| dht.begin(); //Initialize the DHT11 sensor |
| } |
|
|
|
|
| void loop() { |
|
|
| // Reading temperature or humidity takes about 250 milliseconds! |
| // Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor) |
| float h = dht.readHumidity(); |
| // Read temperature as Celsius (the default) |
| float t = dht.readTemperature(); |
| // Read temperature as Fahrenheit (isFahrenheit = true) |
| float f = dht.readTemperature(true); |
|
|
| // Check if any reads failed and exit early (to try again). |
| if (isnan(h) || isnan(t) || isnan(f)) { |
| Serial.println("Failed to read from DHT sensor!"); |
| } |
| else |
| { |
| sprintf(payload,"Humidity:%f Temperature:%f'C",h,t); // Create the payload for publishing |
| |
| if(hornbill.publish(TOPIC_NAME,payload) == 0) // Publish the message(Temp and humidity) |
| { |
| Serial.print("Publish Message:"); |
| Serial.println(payload); |
| } |
| else |
| { |
| Serial.println("Publish failed"); |
| } |
| // publish the temp and humidity every 5 seconds. |
| vTaskDelay(5000 / portTICK_RATE_MS); |
| } |
| } |
Temp and Humidity logged to AWS IOT server.
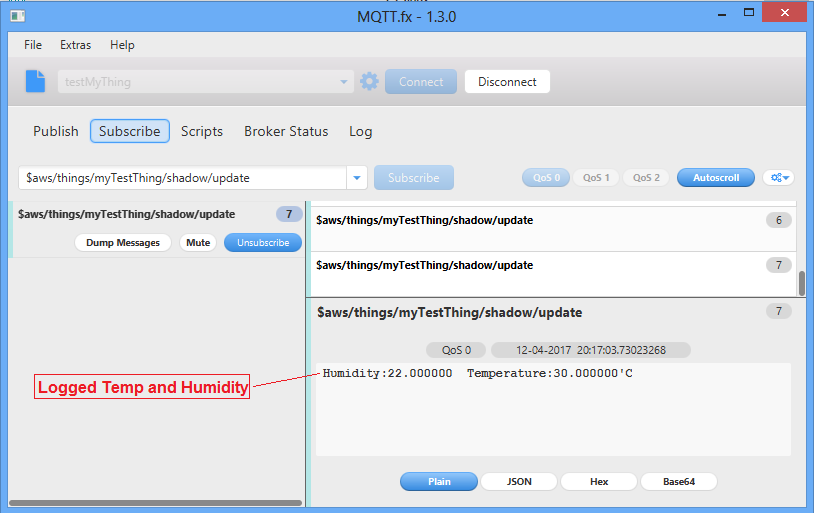
Download
Download the Arduino ESP32 AWS IOT lib and examples form
this link.
0 comentarios:
Publicar un comentario